Circles Area and Circumference C++ Code
This code will show the Area and Circumference of several circles.
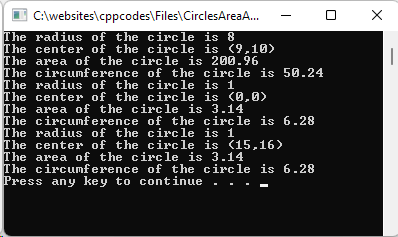
The circles are objects hardcoded in the code. This code makes use of a Circles class.
The Circles Class
The Circles class consists of 4 constructors, a destructor and 4 functions.
The constructors
You can declare a circle object with the following 4 constructors:
Circles circle(); // Will declare a circle object with a radius of 1.
Circles circle2(8); // Will declare a circle object with a radius of 8.
Circles circle3(8, 1, 2); // Will declare a circle object with a radius of 8, and its center coordinates will be x=1 and y=2.
Circles circle4(1, 2); // Will declare a circle object with a radius of 1. Its center coordinates will be x=1 and y=2.
The functions
printCircleStats()
This function will print the current values of a circle object:
Circles circle();
circle.printCircleStats();
// The radius of the circle is 1
// The center of the circle is (0,0)
SetCenter(x, y)
This function will set the center of a circle object:
Circles circle();
sphere.setCenter(9, 10);
circle.printCircleStats();
// The radius of the circle is 1
// The center of the circle is (9,10)
findArea()
This function will find and return the area of the circle:
Circles circle(8);
cout << "The area of the circle is " << sphere2.findArea() << endl;
// The area of the circle is 200.96
findCircumference()
This function will find and return the circumference of the circle:
Circles circle(8);
cout << "The circumference of the circle is " << sphere.findCircumference() << endl;
// The circumference of the circle is 50.24
To change the hardcoded objects in this code, simply alter the constructors and the setCenter function. To create a new circle object, use one of the above constructors.